This blog was originally posted on JavaScript in Plain English.
The ol-kit is a minimally-complicated open-source React and OpenLayers mapping component toolkit. It hits the perfect trifecta of component libraries — easy, good, and cheap. Most only achieve two of those three qualities, but ol-kit accomplishes this by:
- abstracting OpenLayers into familiar-looking React components and utility functions (easy)
- providing a versatile, flexible, and tested package (good), and
- being open source (free = as cheap as it gets).
Add To Existing Apps
Add @bayer/ol-kit and its peer dependencies to your app.
npm i @bayer/ol-kit ol react react-dom styled-components @material-ui/core @material-ui/icons @material-ui/styles --save
Then use the Map component to render a simple map.
import React from 'react'
import { Map } from '@bayer/ol-kit'
const myComponent = () =><Map />
export default myComponent
It’s that easy!
Bootstrap New Apps
Based on the create-react-app bootstrapper, ol-kit offers a one-line bootstrap command (it’ll take a minute or two to run).
npx @bayer/ol-kit create-map
You’ll need to have Node.js installed on your machine for this to work.
Load Map Data
Looking at a map isn’t very useful if there’s no data on it. ol-kit comes with a data loader that can handle most different types of data: GeoJSON, KML, shapefiles, vector tiles, etc.
import React from 'react'
import { Map, loadDataLayer } from '@bayer/ol-kit'
const App = () => {
const onMapInit = async map => {
// nice to have map set on the window while debugging
window.map = map
// find a geojson or kml dataset (url or file) to load on the map
const data = {
url: 'https://data.nasa.gov/api/geospatial/7zbq-j77a?method=export&format=KML',
id: 'world_country_boundaries',
name: 'World Country Boundaries'
}
const dataLayer = await loadDataLayer(map, data.url)
// set the title and id on the layer to show in LayerPanel
dataLayer.set('title', data.name)
dataLayer.set('id', data.name)
}
return <Map onMapInit={this.onMapInit} fullScreen />
}
export default App
Note the onMapInit callback. This is an async callback that will block the map (and other children) from rendering until the promise is resolved, to help prevent other components that might depend on that data from crashing the app.
If you want some unique data to display on the map, check out these free maintained data sources.
Add Map Controls

There are a few UI components that add utility to your map, and the most basic of these are the map controls. Include them on your map by adding the <Controls /> component inside of Map. The actual OpenLayers map instance is passed down via context to all the children of Map, so it does not need to be passed down manually.
import React from 'react'
import { Map, Controls } from '@bayer/ol-kit'
const App = () => {
return (
<Map>
<Controls />
</Map>
)
}
export default App
The default OpenLayers zoom controls are much uglier and far less useful.
Change Basemaps
For this, you have two options: 1. a prebuilt basemap switcher, or 2. a utility function. First, the component option:
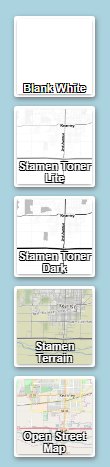
import React from 'react'
import { Map, BasemapContainer } from '@bayer/ol-kit'
const App = () => {
return (
<Map>
<BasemapContainer />
</Map>
)
}
export default App
Or, use the utility:
import React from 'react'
import { Map, loadBasemapLayer } from '@bayer/ol-kit'
const App = () => {
const onMapInit = async map => {
loadBasemapLayer(map, stamenTonerLite')
}
return <Map onMapInit={this.onMapInit} fullScreen />
}
export default App
Enable Popups

A popup is an easy way to provide contextual information on a per-feature basis. The ol-kit allows you to use the default Popup (shown above) that displays all the information on the feature, along with customizable actions, or you can use the PopupBase to build your own, fully-custom popup.
Here’s how to include the popup on your map:
import React from 'react'
import { Map, Popup } from '@bayer/ol-kit'
const App = () => {
return (
<Map>
<Popup />
</Map>
)
}
export default App
Takeaways
The ol-kit offers a lot of customizable components and tools while maintaining a plug-and-play level of ease-of-use. This briefly touches on some of their plug-and-play components; feel free to subscribe to keep up-to-date on more complex implementations.
Here’s the code for the demo app that includes Map, Popup, BasemapContainer, Controls, and a few more cool things.
import React from 'react'
import {
Map,
BasemapContainer,
ContextMenu,
Controls,
LayerPanel,
Popup,
loadDataLayer
} from '@bayer/ol-kit'
const App = () => {
const onMapInit = async map => {
console.log('we got a map!', map)
// nice to have map set on the window while debugging
window.map = map
// find a geojson or kml dataset (url or file) to load on the map
const data = 'https://data.nasa.gov/api/geospatial/7zbq-j77a?method=export&format=KML'
const dataLayer = await loadDataLayer(map, data)
// set the title on the layer to show in LayerPanel
dataLayer.set('title', 'NASA Data')
}
return (
<Map onMapInit={this.onMapInit} fullScreen>
<BasemapContainer />
<ContextMenu />
<Controls />
<LayerPanel />
<Popup />
</Map>
)
}
export default App
Again, check the ol-kit documentation for more information — feel free to reach out with any questions.
Thank you for reading.
And read the next post on how to Add a Map with a Point to Your Website!